본 포스팅은 C++로 시작하는 객체지향 프로그래밍 책을 바탕으로 작성하였습니다.
C++로 시작하는 객체지향 프로그래밍
『C++로 시작하는 객체지향 프로그래밍』은 구문보다는 문제 해결에 중점을 두는 문제 구동 방식을 사용한 프로그래밍에 대해 가르치고 있다. 여러 가지 상황에서 문제를 야기한 개념을 사용함
book.naver.com
key point
- 키보드로부터 입력 값을 읽는다는 것은 프로그램이 사용자로부터 입력을 받을 수 있다는 것이다.
- 식별자는 프로그램에서 변수나 함수와 같은 요소를 확인하는 이름을 말한다.
- 변수는 프로그램에서 변경될 수 있는 값을 표시하기 위해 사용한다.
- cin 객체와 스트림 추출 연산자(>>, streamextraction operator)를 사용하면 콘솔로부터 입력을 받아들일 수 있다.
- 변수를 선언하는 것은 컴파일러에 변수에 저장되는 데이터의 유형이 무엇인지를 알려주는 것이다.
- 함수에서 선언된 변수는 값이 할당되어야 한다. 그렇지 않으면 변수는 초기화되지 않았다고 하며, 변수의 값은 예측 불가능한 값이 된다.
- 이름 상수(named constant) 또는 간단히 상수(constant)는 변하지 않는 영구적인 데이터를 나타낸다.
- 이름 상수는 const 키워드를 사용하여 선언된다.
- C++에는 여러 정밀도의 실수를 표현하는 실수형(float, double, long double)이 있다.
- 정수의 (/) 연산은 정수 값의 결과를 얻는다.
- % 연산자는 정수형에만 가능하다.
check point
2.1
double area = 5.2;
cout << "area"; //area
cout << area; //5.2
2.2.
int a;
cin >> a;
double b;
cin >> b;
2.3
2 * 2.5 = 5.0
2.4 식별자는 프로그램에서 변수나 함수와 같은 요소를 확인하는 이름을 말한다.
2.5 변수는 프로그램에서 변경될 수 있는 값을 표시하기 위해서 사용된다.
2.6 대입문은 변수에 값을 지정하는 것이며, C++ 에서 수식으로 사용된다.
2.7 이름 상수(named constant)를 사용할 때의 이점.
(1) 같은 수치 값을 반복해서 입력할 필요가 없이 간단히 상수 이름을 써 주면 된다.
(2) 상수 값을 변경하고자 할 때 소스코드에서 상수가 선언된 한 곳만 수정해 주면 된다.
(3) 의미에 맞게 상수 이름을 지정해 줌으로써 프로그램의 가독성이 높아진다.
2.8
#include <iostream>
using namespace std;
int main()
{
double miles = 20;
double KILOMETERS_PER_MILE = 1.609;
double kilometers = miles * KILOMETERS_PER_MILE;
cout << kilometers << endl;
return 0;
}
//32.18
2.12
#include <iostream>
using namespace std;
int main()
{
cout << 56 % 6 << endl;
cout << 78 % 4 << endl;
cout << 34 % 5 << endl;
cout << 34 % 15 << endl;
cout << 5 % 1 << endl;
cout << 1 % 5 << endl;
return 0;
}
//2
//2
//4
//4
//0
//1
2.13
#include <iostream>
using namespace std;
int main()
{
cout << (2 + 100) % 7 << endl;
return 0;
}
//4
//즉, 목요일이다
2.19
a = 4.0 / (3 * (r + 34)) - 9 * ( a + b * c) + ( 3 + d * ( 2 + a ) / ( a + b * d ))
b = 5.5 * pow(r + 2.5, 2.5 + t)
list
2.1. 원의 면적을 구하는 간단한 프로그램 작성
#include <iostream>
using namespace std;
int main()
{
double radius;
double area;
//1단계: 반지름 읽기
radius = 20;
//2단계: 면적 계산
area = radius * radius * 3.14159;
//3단계: 면적 출력
cout << "The area is " << area << endl;
return 0;
}
2.2. 키보드로부터 입력 값 읽기
#include <iostream>
using namespace std;
int main()
{
double radius;
cout << "Enter a radius: ";
cin >> radius;
//2단계: 면적 계산
double area = radius * radius * 3.14159;
//3단계: 면적 출력
cout << "The area is " << area << endl;
return 0;
}
2.3. 3개의 수를 읽고 그애 대한 평균 출력
#include <iostream>
using namespace std;
int main()
{
//사용자가 3개의 수를 입력하도록 함
double number1, number2, number3;
cout << "Enter three number: ";
cin >> number1 >> number2 >> number3;
//평균 계산
double average = (number1 + number2 + number3) / 3;
//결과 출력
cout << "The average of" << number1 << " " << number2 << " " << number3 << " is " << average << endl;
return 0;
}
2.5. LimitsDemo.cpp (int)
#include <iostream>
#include <limits>
//visual C++ 외에는 <climits> 또는 <cfloat>
using namespace std;
int main()
{
cout << "INT_MIN" << INT_MIN << endl;
cout << "INT_MAX" << INT_MAX;
return 0;
}
2.6. SizeDemo.cpp (int)
#include <iostream>
#include <limits>
using namespace std;
int main()
{
//int 형에 할당된 바이트의 크기 반환
cout << "The size of int: " << sizeof(int) << "bytes" << endl;
//변수 area 에 할당된 바이트의 크기
double area = 5.4;
cout << "The size of variable area: " << sizeof(area) << "bytes" << endl;
return 0;
}
//The size of int: 4bytes
//The size of variable area: 8bytes
2.7. DisplayTime.cpp
#include <iostream>
using namespace std;
int main()
{
int seconds;
cin >> seconds;
int minutes = seconds / 60;
int remainMinutes = seconds % 60;
cout << minutes << "분 " << remainMinutes << "초 입니다." << endl;
return 0;
}
//500
//8분 20초
2.9 현재 시각 표시하기 (GMT 기준)
#include <iostream>
#include <ctime>
using namespace std;
int main()
{
// 1. 1970년 1월 1일 자정 이후의 초 값 계산
int totalSeconds = time(0);
//2. 현재 시간의 초 값
int nowSeconds = totalSeconds % 60;
// 3. 전체 분 값
int totalMinutes = totalSeconds / 60;
// 4. 현재 시간의 분 값
int nowMinutes = totalMinutes % 60;
// 5. 전체 시간
int totalHours = totalMinutes / 60;
// 6. 현재 시간
int nowHours = totalHours % 24;
cout << nowHours << ":" << nowMinutes << ":" << nowSeconds << endl;
return 0;
}
//13:16:4
Quiz
틀린 퀴즈 문제 모음집.
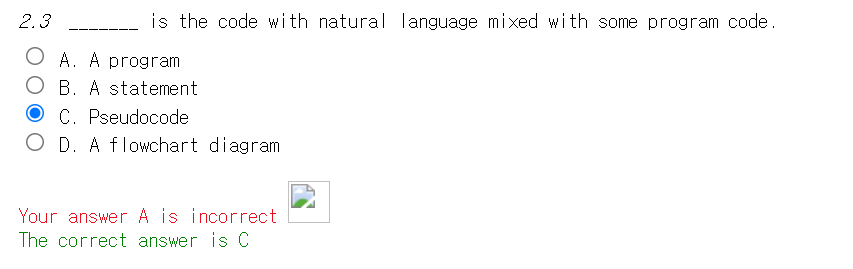
Pseudocode is the code with natural language mixed with some program code.
* pseudocode is a plain language description of the steps in an algorithm or another system.
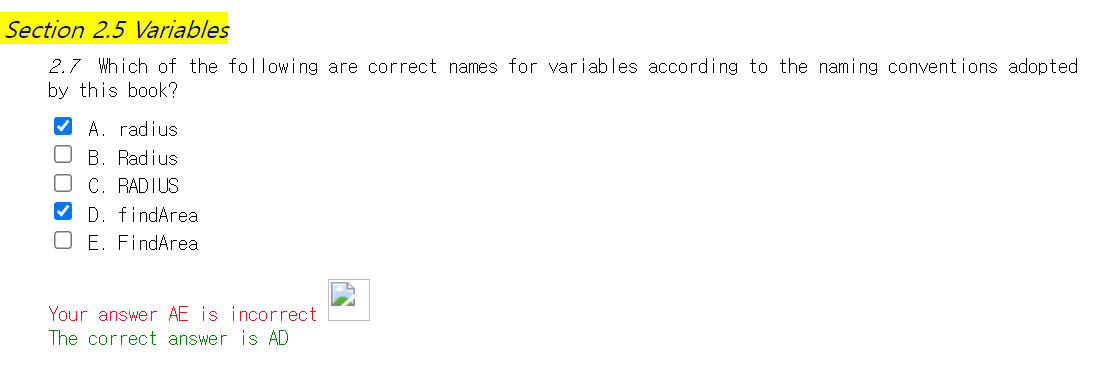
관습적으로 변수는 소문자로 작성하며, 여러 단어가 있을 때는 서로 연결하고 단어의 첫 문자만 대문자로 한다. 단, 첫 단어는 소문자로 한다.
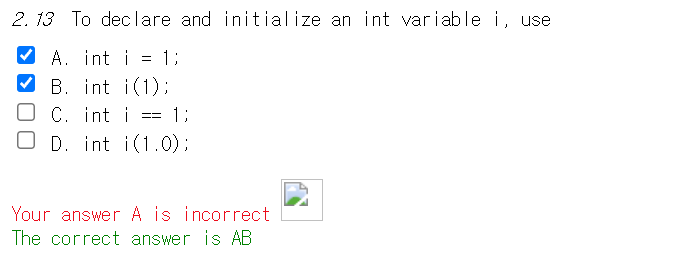
int i(1); 이것도 가능하다고 함.

관습상 상수는 대문자로 작성한다.
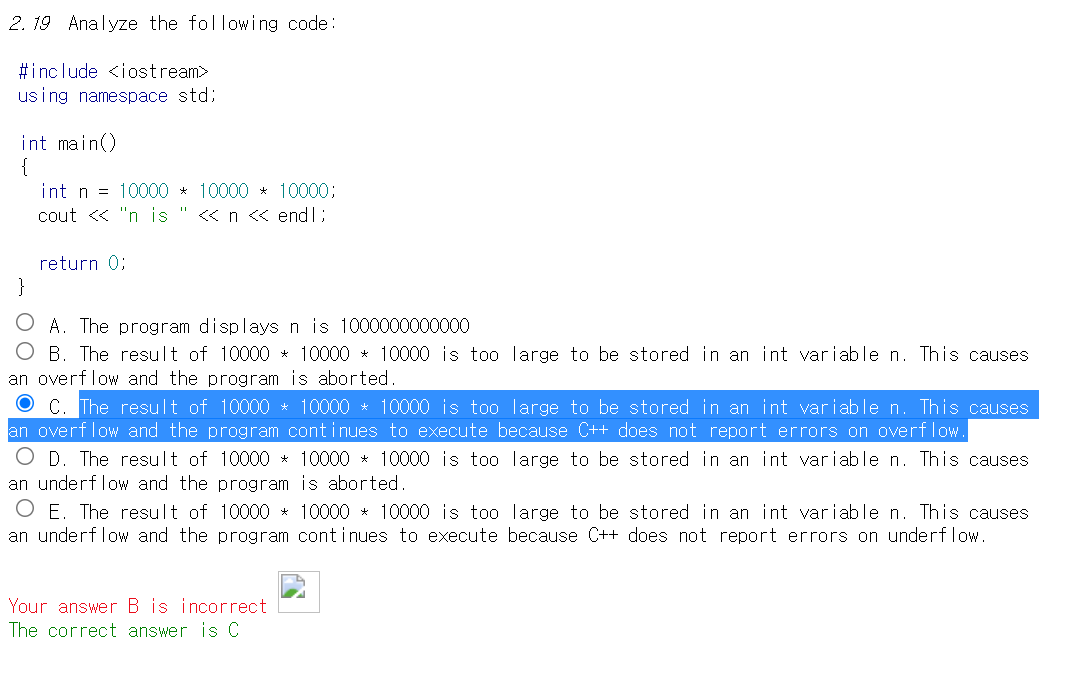
The result of 10000 * 10000 * 10000 is too large to be stored in an int variable n. This causes an overflow and the program continues to execute because C++ does not report errors on overflow.

The program has a syntax error, because 09 is an incorrect literal value.

정수의 (/) 연산은 정수 값의 결과를 얻는다.
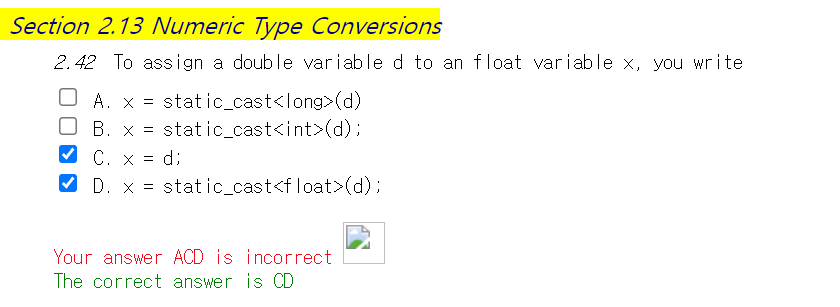
유형확대, 유형축소
실수는 명시적 형변환을 사용하여 정수로 변환될 수 있다.
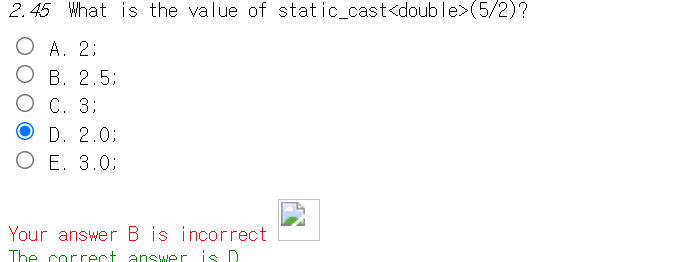
정수의 (/) 연산은 정수 값의 결과를 얻는다. + double
프로그래밍 실습
2.1 섭씨를 화씨로 출력
#include <iostream>
using namespace std;
int main()
{
double celsius;
cout << "Enter a degree in Celsius: ";
cin >> celsius;
double fahrenheit = (9.0 / 5) * celsius + 32;
cout << celsius << " Celsius is " << fahrenheit << " Fahrenheit" << endl;
return 0;
}
//Enter a degree in Celsius: 43
//43 Celsius is 109.4 Fahrenheit
2.2 실린더 용적 계산
int main()
{
double radius;
double length;
cout << "Enter the radius and length of a cylinder: ";
cin >> radius >> length;
double area = radius * radius * 3.14159;
double volume = area * length;
cout << "The area is " << area << endl;
cout << "The volume is " << volume << endl;
return 0;
}
//Enter the radius and length of a cylinder: 5.5 12
//The area is 95.0331
//The volume is 1140.4
2.3 피트를 미터로 변환
int main()
{
double feet;
cout << "Enter a value for feet: ";
cin >> feet;
double meters = feet * 0.305;
cout << feet << " feet is " << meters << " meters" << endl;
return 0;
}
//Enter a value for feet: 16.5
//16.5 feet is 5.0325 meters
2.4 파운드를 킬로그램으로 변환
int main()
{
double pound;
cout << "Enter a number in pounds: ";
cin >> pound;
double kilo = pound * 0.454;
cout << pound << " pound is " << kilo << " kilograms" << endl;
return 0;
}
//Enter a number in pounds: 55.5
//55.5 pound is 25.197 kilograms
2.5 금융 문제 : 상여금 계산
int main()
{
double subtotal; //급여 비율
double gratuity; //상여금 비율
cout << "Enter the subtotal and a gratuity rate: ";
cin >> subtotal >> gratuity;
double gra = gratuity / subtotal;
double total = gra + subtotal;
cout << "The gratuity is $" << gra << " and total is " << total << endl;
return 0;
}
//Enter the subtotal and a gratuity rate: 10 15
//The gratuity is $1.5 and total is 11.5
2.6 정수의 자리 수 더하기
int main()
{
int number;
cout << "Enter a number between 0 and 1000: ";
cin >> number;
int digit1 = number % 10;
int digit2 = (number / 10) % 10;
int digit3 = (number / 10) / 10;
int total = digit1 + digit2 + digit3;
cout << "The sum od the digits is " << total << endl;
return 0;
}
//Enter a number between 0 and 1000: 999
//The sum od the digits is 27
2.7 연도 계산
int main()
{
int min;
cout << "Enter the number of minutes: ";
cin >> min;
int hour = min / 60;
int totalday = hour / 24;
int year = totalday / 365;
int day = totalday % 365;
cout << min << " minutes is approximately " << year << " year and " << day << " days" << endl;
return 0;
}
//Enter the number of minutes: 1000000000
//1000000000 minutes is approximately 1902 year and 214 days
2.9 물리학 : 가속도
int main()
{
double v1;
double v0;
double t;
cout << "Enter v0, v1, and t : ";
cin >> v0 >> v1 >> t;
double a = (v1 - v0) / t;
cout << "The average accelation is " << a << endl;
return 0;
}
//Enter v0, v1, and t : 5.5 50.9 4.5
//The average accelation is 10.0889
2.10 과학 : 에너지 계산
int main()
{
//킬로그램 단위로 물의 양 입력
double water;
cout << "Enter the amount of water in kilograms: ";
cin >> water;
double initial;
cout << "Enter the initial temperature: ";
cin >> initial;
double final;
cout << "Enter the final temperature: ";
cin >> final;
double energy = water * (final - initial) * 4184;
cout << "The energy needed is " << energy << endl;
return 0;
}
//Enter the amount of water in kilograms: 55.5
//Enter the initial temperature: 3.5
//Enter the final temperature: 10.5
//The energy needed is 1.62548e+06 //과학적 표기법 확인
//답이 달라서 찾아보다가 정규화인거 눈치챘다.
//– 이라는 코드가 문제에 있었는데 이거는 -(빼기)이다.
//특수문자코드표 참조하길 바란다.
2.11 인구 예측
int main() {
int putyear;
cout << "Enter the number of years: ";
cin >> putyear;
const int people = 312032486;
double yearplus = ((1.0 / 7.0) * 60 * 60 * 24 * 365) + ((1.0 / 45.0) * 60 * 60 * 24 * 365);
double yearminus = ((1.0 / 13.0) * 60 * 60 * 24 * 365);
int year = yearplus - yearminus;
cout << "The population in " << putyear << " years is " << (putyear * year) + people << endl;
return 0;
}
//Enter the number of years: 5
//The population in 5 years is 325932966
//내가 잘 계산한 것 같은데 샘플결과랑 4정도 차이가 난다.
//뭐가 문제인지 모르겠다.
//너무 오래 붙잡고 있었다.
2.12 물리학 : 활주 길이 계산
int main()
{
//v가 이륙속도 a가 가속도
double v, a;
cout << "비행기의 이륙속도와 가속도를 입력하세요 : ";
cin >> v >> a;
double length = pow(v, 2) / (2 * a);
cout << "비행기가 이륙하기 위한 최고 활주 길이는 " << length << endl;
return 0;
}
//비행기의 이륙속도와 가속도를 입력하세요 : 60 3.5
//비행기가 이륙하기 위한 최고 활주 길이는 514.286
2.15 기하학 : 두 점 간 거리
#include <iostream>
#include <iomainip>
using namespace std;
int main()
{
double x1;
double x2;
double y1;
double y2;
cout << "Enter x1 and y1: ";
cin >> x1 >> y1;
cout << "Enter x2 and y2: ";
cin >> x2 >> y2;
double total = pow((x2 - x1)*(x2 - x1) + (y2 - y1) * (y2 - y1), 0.5);
cout << "The distance between the two points is " << setprecision(16) << total << endl;
return 0;
}
//Enter x1 and y1: 1.5 -3.4
//Enter x2 and y2: 4 5
//The distance between the two points is 8.764131445842194
2.16 기하학 : 육각형의 면적
int main()
{
double side;
cout << "Enter the side: ";
cin >> side;
double hexagon = ((3 * pow(3, 0.5)) / 2) * pow(side, 2);
cout << "The area of the hexagon is " << hexagon << endl;
return 0;
}
//Enter the side: 5.5
//The area of the hexagon is 78.5918
2.18 표 출력
int main()
{
int a, b;
cout << "a " << "b " << "pow(a, b) " << endl;
a = 1, b = 2;
cout << a << " " << b << " " << pow(a, b) << endl;
a = 2, b = 3;
cout << a << " " << b << " " << pow(a, b) << endl;
a = 3, b = 4;
cout << a << " " << b << " " << pow(a, b) << endl;
a = 4, b = 5;
cout << a << " " << b << " " << pow(a, b) << endl;
a = 5, b = 6;
cout << a << " " << b << " " << pow(a, b) << endl;
return 0;
}
//a b pow(a, b)
//1 2 1
//2 3 8
//3 4 81
//4 5 1024
//5 6 15625
2.19 기하학 : 삼각형의 면적
int main()
{
double x1, y1;
cout << "(x1, y1)을 입력하세요 : " << endl;
cin >> x1 >> y1;
double x2, y2;
cout << "(x2, y2)을 입력하세요 : " << endl;
cin >> x2 >> y2;
double x3, y3;
cout << "(x3, y3)을 입력하세요 : " << endl;
cin >> x3 >> y3;
double side1 = pow(((x1 - x2) * (x1 - x2) + (y1 - y2) * (y1 - y2)), 0.5);
double side2 = pow(((x2 - x3) * (x2 - x3) + (y2 - y3) * (y2 - y3)), 0.5);
double side3 = pow(((x3 - x1) * (x3 - x1) + (y3 - y1) * (y3 - y1)), 0.5);
double s = ((side1 + side2 + side3)) / 2;
double area = pow(s * (s - side1) * (s - side2) * (s - side3), 0.5);
cout << "삼각형의 면적은 " << area << endl;
return 0;
}
//(x1, y1)을 입력하세요 :
//1.5 -3.4
//(x2, y2)을 입력하세요 :
//4.6 5
//(x3, y3)을 입력하세요 :
//9.5 -3.4
//삼각형의 면적은 33.6
'Language > C++' 카테고리의 다른 글
[C++_제 5장] 반복문 (0) | 2021.10.09 |
---|---|
[C++_제 4장] 수학 함수, 문자, 문자열 (0) | 2021.10.09 |
[C++_제 3장] 선택문 (0) | 2021.10.03 |
[C++_백준] 입출력과 사칙연산 ( 10171, 10172, 1000, 1001, 10998, 1008, 10869, 10430, 2588) (0) | 2021.10.01 |
[C++_제 1장] 컴퓨터, 프로그램 및 C++ 입문 (0) | 2021.09.22 |