본 포스팅은 쉽게 배우는 자바 프로그래밍 교재를 바탕으로 작성되었습니다.
Chapter 07장 인터페이스와 특수 클래스
📍도전 과제
01. count() 추상 메서드를 가진 Countable 인터페이스를 Bird 클래스와 Tree 클래스로 각각 구현해 보자.
클래스
public interface Countable {
void count();
}
class Bird implements Countable {
String name;
public Bird(String name) {
this.name = name;
}
@Override
public void count() {
System.out.println(name + "가 2마리 있다.");
}
void fly() {};
}
class Tree implements Countable {
String name;
public Tree(String name) {
this.name = name;
}
@Override
public void count() {
System.out.println(name + "가 5그루 있다.");
}
void ripen() {};
}
<Test 코드>
public class CountableTest {
public static void main(String[] args) {
Countable[] m = {new Bird("뻐꾸기"), new Bird("독수리"),
new Tree("사과나무"), new Tree("밤나무")} ;
for (Countable e : m)
e.count();
}
}
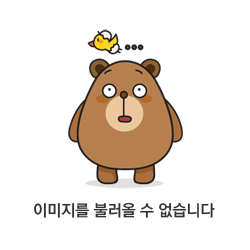
02. 타입에 따라 다른 메서드를 호출하는 다형성을 테스트하는 코드를 테스트 프로그램에서 추가해 보자.
이 문제가 가장 어려웠던 것 같다. 상속관계가 아니라서 instanceof가 안될 줄 알고 어떻게 해당 타입인지 코드를 짜는게 어려웠다. 구현 관계여도 instanceof는 사용할 수 있고, 저렇게 타입변환을 해서 메서드를 호출하면 된다.
public class CountableTest {
public static void main(String[] args) {
Countable[] m = {new Bird("뻐꾸기"), new Bird("독수리"),
new Tree("사과나무"), new Tree("밤나무")};
for (Countable e : m)
e.count();
for (int i = 0; i < m.length; i++) {
if (m[i] instanceof Bird)
((Bird) m[i]).fly();
else if (m[i] instanceof Tree)
((Tree) m[i]).ripen();
}
}
}
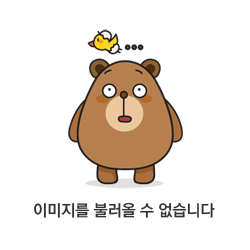
03. 다음 그림과 같이 Countable 인터페이스를 추상 클래스로 바꾸고 소유자 필드도 추가해 보자.
abstract class Countable {
protected String name;
protected int num;
public Countable(String name, int num) {
this.name = name;
this.num = num;
}
abstract void count();
}
class Bird extends Countable {
public Bird(String name, int num) {
super(name, num);
}
@Override
void count() {
System.out.println(name + "가 " + num + "마리 있다.");
}
void fly() {
System.out.println(num + "마리 " + name + "가 날아간다.");
}
}
class Tree extends Countable {
public Tree(String name, int num) {
super(name, num);
}
@Override
void count() {
System.out.println(name + "가 " + num + "그루 있다.");
}
void ripen() {
System.out.println(num + "그루 " + name + "에 열매가 잘 익었다.");
}
}
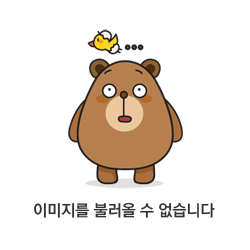
📍프로그래밍 문제
01번. 추상 클래스도 생성자를 가질 수 있다. 다음 표와 같이 추상 클래스와 구현 클래스를 작성한 후 아래 테스트 프로그램을 실행하라. 단, 추상 클래스와 구현 클래스의 생성자는 모든 필드를 초기화한다.
public class AbstractTest {
public static void main(String[] args) {
Concrete c = new Concrete(100, 50);
c.show();
}
}
abstract class Abstract {
int i;
public Abstract(int i) {
this.i = i;
}
abstract void show();
}
class Concrete extends Abstract {
int j;
public Concrete(int i, int j) {
super(i);
this.j = j;
}
@Override
void show() {
System.out.println("i = " + i + ", j = " + j);
}
}
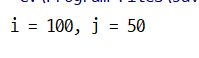
02. 다음과 같이 2개의 인터페이스가 있다. 이 2개의 인터페이스를 모두 사용하는 클래스가 자주 발생한다. 하나의 인터페이스로 통합된 Delicious 인터페이스를 작성하라.
interface Delicious extends Edible, Sweetable{
}
interface Edible {
void eat();
}
interface Sweetable {
void sweet();
}
03. 가격 순서대로 정렬할 수 있는 Book 클래스와 다음 실행 결과가 나타나도록 테스트 프로그램을 작성하라, Book 클래스에는 int 타입의 price 필드가 있으며, 생성자와 필요한 메서드를 포함한다. 또 테스트 프로그램은 3개의 Book 객체로 구성된 Book 배열을 사용해 가격 순서대로 정렬한 후 출력한다,
⭐ 정렬때문에 어려웠던 문제이다. 결국은 인터넷 찾아보면서 답을 알아냈다. 정렬만 하면 알아서 해줄 줄 알았는데, 내가 코딩을 해야되는 거였다. 무엇을 정렬할지 정의해주기
정말 착하게 설명해주셨다. 이 블로그 읽으면 이해 완료
https://tooo1.tistory.com/104?category=936545
[쉽게 배우는 자바 프로그래밍] 7장 : 3번 - JAVA[자바]
⊙ 문제 가격 순서대로 정렬할 수 있는 Book 클래스와 다음 실행 결과가 나타나도록 테스트 프로그램을 작성하시오. Book 클래스에는 int 타입의 price 필드가 있으며, 생성자와 필요한 메서드를 포
tooo1.tistory.com
<테스트 클래스>
import java.util.Arrays;
public class BookTest {
public static void main(String[] args) {
Book[] books = { new Book(15000), new Book(50000), new Book(20000) };
System.out.println("정렬 전");
for (Book b : books)
System.out.println(b.toString());
Arrays.sort(books);
System.out.println("정렬 후");
for (Book b : books)
System.out.println(b.toString());
}
}
<클래스 방법1>
class Book implements Comparable {
int price;
public Book(int price) {
this.price = price;
}
@Override
public int compareTo(Object o) {
Book book = (Book) o;
/*if (this.price < book.price)
return -1;
else if (this.price > book.price)
return 1; //자리변경
else
return 0;*/
return this.price - book.price;
}
@Override
public String toString() {
return "Book [" +
"price=" + price +
']';
}
}
<방법 2> - record 사용
record Books(int price) implements Comparable<Books>{
@Override
public int compareTo(Books b) {
return price - b.price;
}
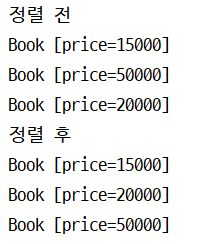
04. Talkable 인터페이스 talk() 메서드 하나만 포함한다. Korean 클래스와 American 클래스는 Talkable 구현 클래스이다. 다음 테스트 프로그램과 실행 결과를 참고해 Talkable 인터페이스와 Korean 클래스, American 클래스를 구현하고, 테스트 프로그램도 완성하라.
public class TalkableTest {
static void speak(Talkable talkable) {
talkable.talk();
}
public static void main(String[] args) {
speak(new Korean());
speak(new American());
}
}
interface Talkable {
void talk();
}
class Korean implements Talkable {
@Override
public void talk() {
System.out.println("한국인입니다. 안녕하세요~");
}
}
class American implements Talkable {
@Override
public void talk() {
System.out.println("미국인입니다. Hi~");
}
}
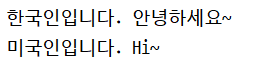
05. 다음 표와 같은 멤버를 가진 Controller 추상 클래스가 있다. TV와 Radio 클래스는 Controller의 구현 클래스이다. Controller, TV, Radio 클래스를 작성하라. 그리고 ControllerTest 프로그램으로 테스트하라.
구현클래스에서 power를 this.power = power로 해서 매개변수로 받은 boolean값이 제대로 작동하지 못했다. super(boolean)으로 받아야지 추상클래스로 가서 그걸 show() 함수로 넘겨줄 수 있는데,,, 나는 아직 많이 부족한가 보다.
public class ControllerTest {
public static void main(String[] args) {
Controller[] c = { new TV(false), new Radio(true) };
for (Controller controller : c)
controller.show();
}
}
abstract class Controller {
boolean power;
public Controller(boolean power) {
this.power = power;
}
abstract String getName();
void show() {
if (power)
System.out.println(getName() + "가 켜졌습니다.");
else
System.out.println(getName() + "가 꺼졌습니다.");
};
}
class TV extends Controller {
public TV(boolean power) {
super(power);
}
@Override
String getName() {
return "TV";
}
}
class Radio extends Controller {
public Radio(boolean power) {
super(power);
}
@Override
String getName() {
return "라디오";
}
}
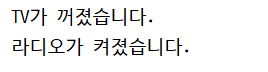
06. 다음과 같은 테스트 프로그램을 실행하고자 한다. Human 인터페이스, Worker 클래스에 수정할 부분이 있으면 수정하고, Student 클래스도 작성하라.
public class HumanTest {
public static void main(String[] args) {
Human.echo();
Student s = new Student(20);
s.print();
s.eat();
Human p = new Worker();
p.print();
p.eat();
}
}
interface Human {
void eat();
void print();
static void echo() {
System.out.println("야~호~!!1");
}
}
class Worker implements Human {
public void eat() {
System.out.println("빵을 먹습니다.");
}
@Override
public void print() {
System.out.println("인간입니다.");
}
}
class Student implements Human {
int age;
public Student(int age) {
this.age = age;
}
@Override
public void eat() {
System.out.println("도시락을 먹습니다.");
}
@Override
public void print() {
System.out.println(age + "세의 학생입니다.");
}
}
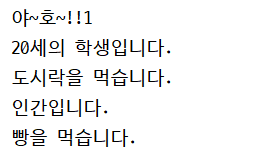
07. Flyable 인터페이스와 테스트 프로그램을 실행한 결과가 다음과 같다. Flyable 인터페이스를 지역 클래스로 이용하는 테스트 프로그램을 완성하라.
문제 뜻이 잘 이해가 가지 않았다. 그냥 컴파일러가 해주는 대로 따라가면 된다.
interface Flyable {
void speed();
void height();
}
public class FlyableTest {
public static void main(String[] args) {
Flyable f = new Flyable() {
@Override
public void speed() {
System.out.println("속도");
}
@Override
public void height() {
System.out.println("높이");
}
};
f.speed();
f.height();
}
}

08. 다음과 같은 Echoer 클래스와 테스트 프로그램, 실행 결과가 있다. 테스트 프로그램은 Echoer 클래스를 이용해 키보드로 입력한 메시지를 화면에 출력한다. 테스트 프로그램을 완성하라.
import java.util.Scanner;
public class EchoerTest {
public static void main(String[] args) {
Scanner in = new Scanner(System.in);
Echoer e = new Echoer() {
@Override
void echo() {
String a = in.nextLine();
while(!a.equals("끝")) {
System.out.println(a);
a = in.nextLine();
}
System.out.println(a);
}
};
e.start();
e.echo();
e.stop();
}
}
abstract class Echoer {
void start() {
System.out.println("시작합니다.");
}
abstract void echo();
void stop() {
System.out.println("종료합니다.");
}
}
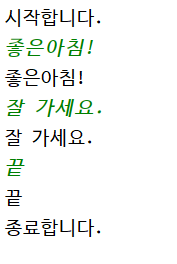
'Language > JAVA' 카테고리의 다른 글
[JAVA] 백준_입출력과 사칙연산(2557, 1000, 10869, 10926, 18108, 3003, 10430) (1) | 2023.11.09 |
---|---|
[JAVA] 쉽게 배우는 자바 프로그래밍_8장 기본 패키지 (0) | 2022.06.13 |
[JAVA] 쉽게 배우는 자바 프로그래밍_6장 상속 (0) | 2022.06.11 |
[JAVA] 쉽게 배우는 자바 프로그래밍_5장 문자열, 배열, 열거 타입 (0) | 2022.04.25 |
[JAVA] 쉽게 배우는 자바 프로그래밍_03장 제어문과 메서드 (0) | 2022.04.24 |